[DEPRECATED] Build Custom Views with Data
Stream data as callbacks to native apps
One of the more frequent use cases supported by HyperTrack is live location status streaming to your app user's mobile device.
For example, if you are developing a carpool app, you want to provide a native real-time view of a driver on the way to a pickup destination, with up-to-date location and status with ETA.
To help you build apps where native in-app real-time tracking experience is required, HyperTrack provides you Views SDKs for Android and iOS as building blocks. You can use these building blocks to create real-time location tracking experience in the map layer of your own choosing, with full control of UI/UX while HyperTrack provides you real-time location data updates and infrastructure. This way, you focus on creating compelling app experience without worrying about building location tracking infrastructure.
Views SDKs connect directly to HyperTrack cloud and provide you callbacks you can use in your app to stream real-time data for devices involved in your business workflow. Views SDKs stream devices' locations, status changes, as well as trip updates to your mobile app directly from HyperTrack platform.
:::important
HyperTrack Views SDK on both Android and iOS enable you to deliver real-time location tracking experience without you needing to set up your own servers.
HyperTrack does this directly for you by pushing live subscriptions to your app via Views SDK in real-time at scale.
:::
Live location streaming on Android
The Android Views SDK is used for getting live location and movement data for devices and trips directly to your native Android app. Please follow steps below to learn how to integrate the SDK and get started with its API.
:::note
Currently we do support all of the Android versions starting from API 19 (Android 4.4 Kit Kat)
:::
Add Views SDK
Add following lines to your app build.gradle
file:
// Import the SDK within your repositories block
repositories {
maven {
name 'hypertrack'
url 'http://m2.hypertrack.com'
}
...
}
//Add HyperTrack Views SDK as a dependency
dependencies {
implementation 'com.hypertrack:hypertrack-views:<version>'
...
}
Add Proguard config
If you are using Proguard, add this to the Proguard config:
# To keep line numbers in stack traces
-keepattributes SourceFile,LineNumberTable
##---------------Begin: Amazon client ----------
-keep class com.amazonaws.amplify.** {*;}
-keep class type.** {*;}
-keep class org.apache.commons.logging.** { *; }
-keep class com.amazonaws.javax.xml.transform.sax.* { public *; }
-keep class com.amazonaws.javax.xml.stream.** { *; }
-keep class com.amazonaws.services.**.model.*Exception* { *; }
-keep class org.codehaus.** { *; }
-keepattributes Signature, *Annotation*
-dontwarn javax.xml.stream.events.**
-dontwarn org.codehaus.jackson.**
-dontwarn org.apache.commons.logging.impl.**
-dontwarn org.apache.http.conn.scheme.**
##---------------End: Amazon client ----------
##---------------Begin: Gson ----------
# Gson uses generic type information stored in a class file when working with fields. Proguard
# removes such information by default
-keepattributes Signature
# For using GSON @Expose annotation
-keepattributes *Annotation*
# Gson specific classes
-keep public class com.google.gson.**
-keep class com.google.gson.stream.** { *; }
-dontwarn sun.misc.**
# Application classes that will be serialized/deserialized over Gson
-keep class com.hypertrack.sdk.views.dao.** { *; }
-keep class com.hypertrack.sdk.views.HyperTrackViews { *; }
# Prevent proguard from stripping interface information from TypeAdapterFactory,
# JsonSerializer, JsonDeserializer instances (so they can be used in @JsonAdapter)
-keep class * implements com.google.gson.TypeAdapterFactory
-keep class * implements com.google.gson.JsonSerializer
-keep class * implements com.google.gson.JsonDeserializer
##---------------End: Gson ----------
Instantiate Views SDK
Pass Context
reference to get SDK instance.
HyperTrackViews hypertrackView = HyperTrackViews.getInstance(this, PUBLISHABLE_KEY);
Identify which device to track
For example, your app user a passenger waiting for a pickup. Your passenger app user needs to be able to see driver's device in real-time inside your app. You have a database of drivers, and dispatched an available driver to pick up your passenger. The driver is identified by the device id.
Please see this guide on how to get and store device ids required for your application workflow.
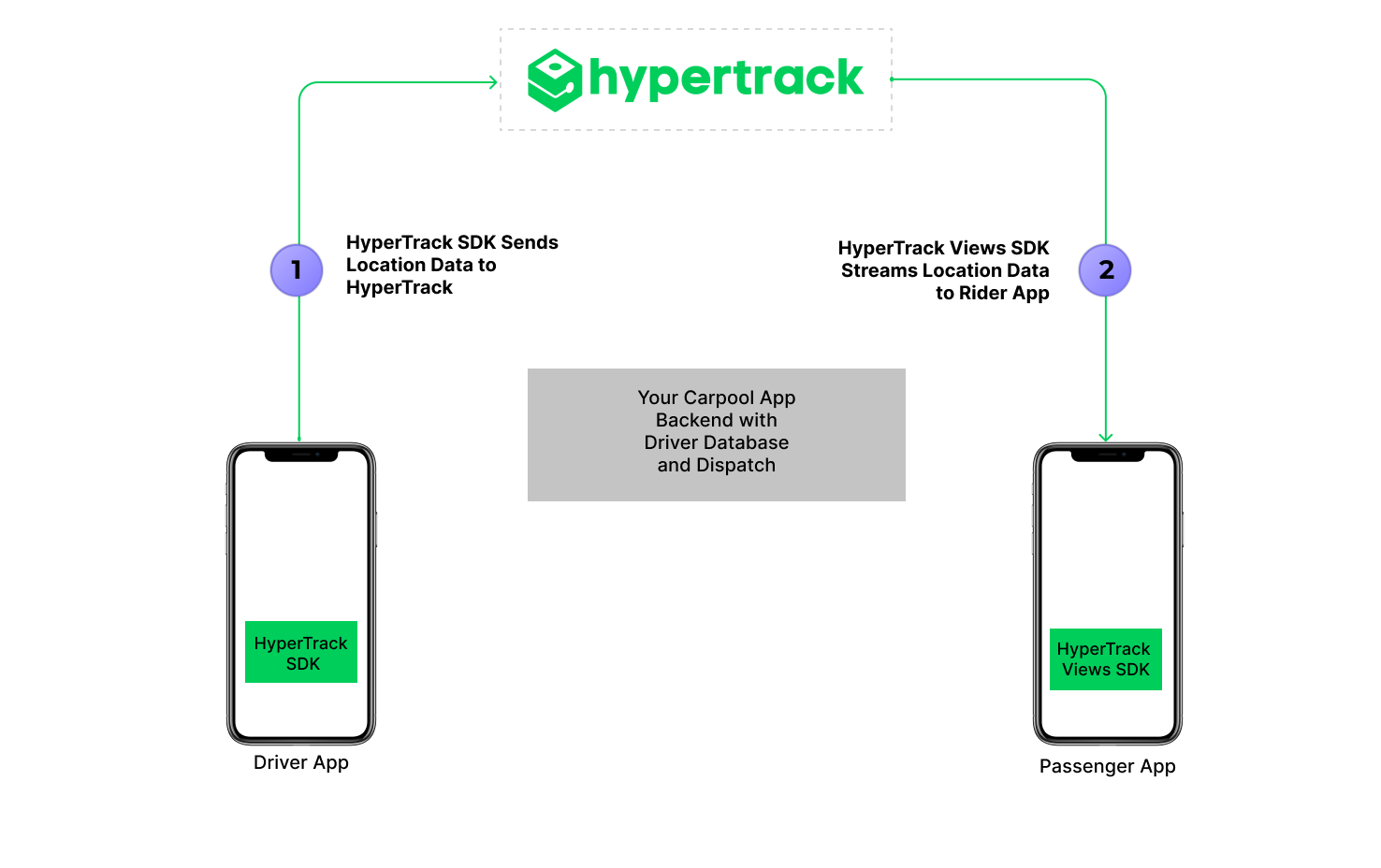
Your driver app user runs a dedicated driver app which integrates HyperTrack SDK on either Android or iOS. The driver's app is on the move and generates location data sent by HyperTrack SDK.
Get current location of tracked device
With the SDK, you can get current status of another's app user device status. For example, once a passenger launches the app, you may call to get location of the driver on the way to a pickup spot.
To do this, get current state of this driver's device, identified by driverDeviceId
as shown in the snippet below:
hypertrackView.getDeviceMovementStatus(
driverDeviceId,
new Consumer<MovementStatus>() {
@Override
public void accept(MovementStatus movementStatus) {
Log.d(TAG, "Got movement status data " + movementStatus);
}
});
In the callback, you'll receive MovementStatus object which describes the current state of the driver's device. This object also contains location that you may able to use to render the current driver location with its status in the passenger's app.
Subscribe to streaming updates to get location data
You can receive device state changes updates as shown in the snippet below. In the example below, you can see the following callback methods:
onLocationUpdateReceived
- gives you the location update for a deviceonBatteryStateUpdateReceived
- battery state change updateonStatusUpdateReceived
- device status changeonTripUpdateReceived
- provides a trip status updateonError
- provides feedback about errors that may occur in streamingonCompleted
- notifies about a successful completion of subscription being stopped
hypertrackView.subscribeToDeviceUpdates(
driverDeviceId,
new DeviceUpdatesHandler() {
@Override
public void onLocationUpdateReceived(@NonNull Location location) {
Log.d(TAG, "onLocationUpdateReceived: " + location);
}
@Override
public void onBatteryStateUpdateReceived(@BatteryState int i) {
Log.d(TAG, "onBatteryStateUpdateReceived: " + i);
}
@Override
public void onStatusUpdateReceived(@NonNull StatusUpdate statusUpdate) {
Log.d(TAG, "onStatusUpdateReceived: " + statusUpdate);
}
@Override
public void onTripUpdateReceived(@NonNull Trip trip) {
Log.d(TAG, "onTripUpdateReceived: " + trip);
}
@Override
public void onError(Exception exception, String deviceId) {
Log.w(TAG, "onError: ", exception);
}
@Override
public void onCompleted(String deviceId) {
Log.d(TAG, "onCompleted: " + deviceId);
}
}
);
Tracking trips with Views SDK
Examples above illustrate how to create a passenger app and use Views SDK to track a driver. You may create a trip for the driver as explained in Track live route and eta to destination guide.
Since each trip created has a device id, you may use this device id to subscribeToDeviceUpdates
. This way, you will get trip status updates, which include ETA, ETA changes, as well as delays via onTripUpdateReceived
callback.
Streaming location data from multiple devices
In use cases where you need to track multiple devices in realtime in the app, you can easily accomplish this goal by subscribing to more than one device.
In the example below, you may execute subscribeToDeviceUpdates
to get streaming updates for driverDeviceId_1
and driverDeviceId_2
.
hypertrackView.subscribeToDeviceUpdates(
driverDeviceId_1,
new DeviceUpdatesHandler() {
@Override
public void onLocationUpdateReceived(@NonNull Location location) {
Log.d(TAG, "onLocationUpdateReceived: " + location);
}
}
hypertrackView.subscribeToDeviceUpdates(
driverDeviceId_2,
new DeviceUpdatesHandler() {
@Override
public void onLocationUpdateReceived(@NonNull Location location) {
Log.d(TAG, "onLocationUpdateReceived: " + location);
}
}
);
Stopping real-time streaming updates in app
Finally, if you wish to stop streaming updates or if the app user exits the screen where this experience is no longer required, you should invoke stopAllUpdates
method in your HyperTrack Views instance.
hypertrackView.stopAllUpdates();
Live location streaming on iOS
The iOS Views SDK is used for getting live location and movement data for devices and trips directly to your native Android app.
Please follow steps below to learn how to integrate the SDK and get started with its API.
Getting started with Views SDK on iOS
Clone HyperTrack Views iOS repository
git clone https://github.com/hypertrack/views-ios.git
cd views-ios
Install Views SDK dependency
Please use CocoaPods dependency manager to install the latest version of the HyperTrackViews library. Using the latest version of CocoaPods is advised.
If you don't have CocoaPods, install it first. Run pod install
inside the cloned directory. After CocoaPods creates the ViewsExample.xcworkspace
workspace file, open it with Xcode.
Set your Publishable Key and Device ID
Open the ViewsExample project inside the workspace and set your Publishable Key and Device ID inside the placeholder in the ViewController.swift
file.
Run the ViewsExample app
Run the app on your phone or simulator and you should see the map with your device on it. You can see the subscription status and full MovementStatus data structure in Console logs inside Xcode (for apps built in DEBUG mode).
Integrate HyperTrackViews library
:::note
HyperTrackViews supports iOS 11.2 and above, using Swift language.
:::
Add HyperTrackViews to your project
We use CocoaPods to distribute the library, you can install it here.
Using command line run pod init
in your project directory to create a Podfile. Put the following code (changing target placeholder to your target name) in the Podfile:
platform :ios, '11.2'
inhibit_all_warnings!
target '<Your app name>' do
use_frameworks!
pod 'HyperTrackViews'
end
Run pod install
. CocoaPods will build the dependencies and create a workspace (.xcworkspace
) for you.
Create an instance
To create an instance of HyperTrackViews, pass it your publishable key:
let hyperTrackViews = HyperTrackViews(publishableKey: publishableKey)
You can initialize the library wherever you want. If reference gets out of scope, library will cancel all subscriptions and network state after itself.
Get current location of tracked device
Please review the above section on how to get a device id for the device that needs to be traceked.
You can get a snapshot of your device movement status with movementStatus(for:completionHandler:)
function:
let cancel = hyperTrackViews.movementStatus(for: "Paste_Your_Device_ID_Here") {
[weak self] result in
guard let self = self else { return }
switch result {
case let .success(movementStatus):
// Update your UI with movementStatus structure
case let .failure(error):
// React to errors
}
}
Update your UI using data from MovementStatus structure.
You can use cancel
function to cancel the request if you want, just run cancel()
to cancel the request.
You can ignore the return value from movementStatus(for:completionHandler:)
by using let _ = moveme...
pattern (The use of the pattern is needed until Apple will fix SR-7297 bug).
Subscribe to streaming updates to get location data
You can get movement status continuously every time tracked device updates its location. This function makes a movementStatus(for:completionHandler:)
call under the hood, so you'll get initial status right away.
let cancel = hyperTrackViews.subscribeToMovementStatusUpdates(
for: driverDeviceId) { [weak self] result in
guard let self = self else { return }
switch result {
case .success(let movementStatus):
// Update your UI with movementStatus structure
case .failure(let error):
// React to subscription errors
}
}
You need to hold on to the cancel()
function until you don't need subscription results.
If this function gets out of scope, subscription will automatically cancel and all network resources and memory will be released. This is useful if subscription is needed only while some view or controller is in the scope.
Tracking trips with Views SDK
Examples above illustrate how to create a passenger app and use Views SDK to track a driver. You may create a trip for the driver as explained in Track live route and eta to destination guide.
Since each trip created has a device id, you may use this device id to subscribeToMovementStatusUpdates
. This way, you will get trip status updates, which include ETA, ETA changes, as well as delays via onTripUpdateReceived
callback.
Streaming location data from multiple devices
In use cases where you need to track multiple devices in realtime in the app, you can easily accomplish this goal by subscribing to more than one device.
In the example below, you may execute subscribeToMovementStatusUpdates
to get streaming updates for driverDeviceId_1
and driverDeviceId_2
.
let cancel = hyperTrackViews.subscribeToMovementStatusUpdates(
for: driverDeviceId_1) { [weak self] result in
guard let self = self else { return }
switch result {
case .success(let movementStatus):
// Update your UI with movementStatus structure
case .failure(let error):
// React to subscription errors
}
}
let cancel = hyperTrackViews.subscribeToMovementStatusUpdates(
for: driverDeviceId_2) { [weak self] result in
guard let self = self else { return }
switch result {
case .success(let movementStatus):
// Update your UI with movementStatus structure
case .failure(let error):
// React to subscription errors
}
}
Special note on Xcode 10.1 Support
If you want to run the project on Xcode 10.1 that doesn't support Swift 5, you need to add this post_install
script at the end of your Podfile. For this project you can copy-paste the following snippet below:
platform :ios, '9.0'
target 'ViewsExample' do
use_frameworks!
pod 'HyperTrackViews'
end
post_install do |installer|
installer.pods_project.targets.each do |target|
if ['HyperTrackViews', 'AWSCore', 'AWSAppSync', 'ReachabilitySwift', 'SQLite.swift'].include? target.name
target.build_configurations.each do |config|
config.build_settings['SWIFT_VERSION'] = '4.2'
end
end
end
end
This script will set Swift 4.2 for all HyperTrackViews dependencies, so you don't need to do this every time.
Then you need to go to the Pods project inside the workspace then Pods > SQLite.swift > standard > Foundation.swift
and change datatypeValue
function to:
public var datatypeValue: Blob {
return withUnsafeBytes { (pointer: UnsafePointer<UInt8>) -> Blob in
return Blob(bytes: pointer, length: count)
}
}
Select Unlock
when Xcode prompts, then build the project. You'll need to change datatypeValue
function every pod reinstall, until this issue is closed.
Rererence
For a full iOS Views SDK reference see iOS Views SDK Reference
Summary
HyperTrack Views SDK for both Android and iOS allow you to stream location data from devices using HyperTrack SDK.
HyperTrack SDKs for for Android and iOS, including Flutter and ReactNative modules, allow you to integrate location tracking. They automatically send location in real-time whenever you use HyperTrack Devices and Trips API to control their location tracking.
With HyperTrack Devices and Trips APIs, HyperTrack SDKs, and HyperTrack Views SDKs you have a full set of tools powered by HyperTrack infrastructure to help you create apps such as carpooling, ridesharing, as well as any other use cases requiring real-time native app tracking experiences. We can't wait to see you focus on what you do best: create and build apps while leaving the worries of location tracking infrastructure and tools to HyperTrack.
Updated 9 months ago